Using the debug() command
Overview
This is a new command added in Nightwatch v2.3.0, which allows users to pause the test at any point (by using .debug()
command as a breakpoint) and use a REPL interface (made available in the terminal) to try out the available Nightwatch commands and assertions and see them get executed against the running browser, in real-time.
While doing that, users can also interact with the browser and use DevTools to debug. The interface also supports multi-line code input and auto-complete feature.
Usage
Note: Please use async/await
while using the .debug()
command, otherwise proper results won't be returned back to the interface.
it('demos debug command', async function(browser) {
await browser.debug();
// with no auto-complete
await browser.debug({preview: false});
// with a timeout of 6000 ms (time for which the interface
// would wait for a result, default is 5500ms).
await browser.debug({timeout: 6000})
});
Example
tests/duckDuckGo.js
describe('duckduckgo debug example', function() {
// function passed as second argument to `it` should be `async`.
it('Search Nightwatch.js and check results', async function(browser) {
await browser
.url('https://duckduckgo.com')
.debug()
.waitForElementVisible('#search_form_input_homepage')
.sendKeys('#search_form_input_homepage', ['Nightwatch.js'])
.click('#search_button_homepage')
.assert.visible('.results--main')
.assert.textContains('.results--main', 'Nightwatch.js');
});
});
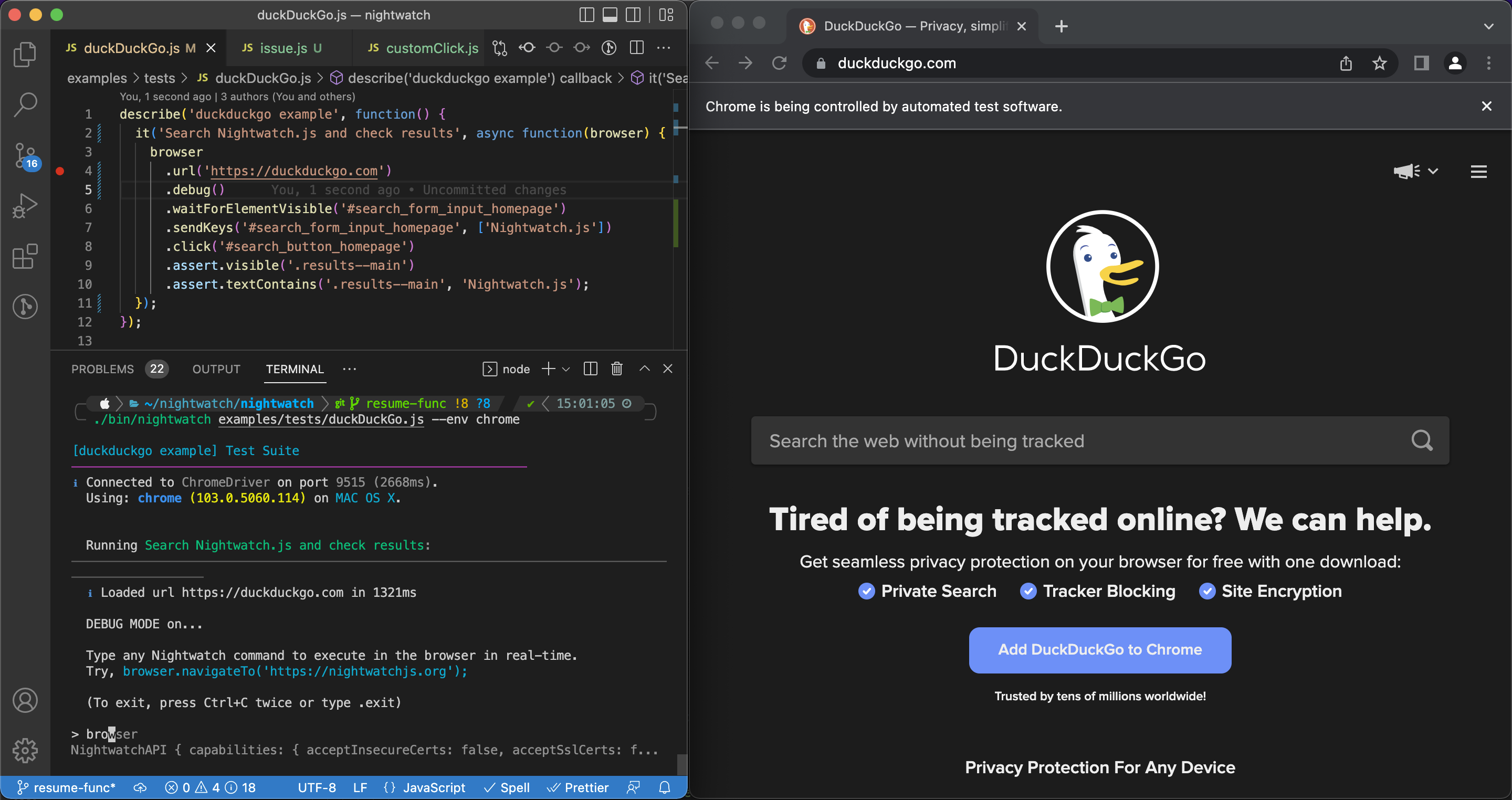