Using the pause() command
Overview
The .pause()
command allows users to pause their test execution, either for a fixed amount of time (by passing the duration in milliseconds as an argument) or for an unrestricted amount of time with an option to resume anytime.
To suspend the text execution for a limited amount of time (e.g. 300ms), write:
it('demos pause command', function(browser) {
// pause for 300 ms
browser.pause(300);
});
To suspend the text execution indefinitely, until resumed, write:
it('demos pause command', function(browser) {
// pause indefinitely, until resumed
browser.pause();
});
Usage
While the .pause(ms)
command is mostly used programmatically to pause the test for a small duration of time before performing the next command, pause()
command (without any argument) can be used while debugging.
While using the pause()
command without any argument, the following operations are available:
- resume the test normally from the point where it was left off
- step over to the next command/assertion and pause again
- exit from the test run
tests/duckDuckGo.js
describe('duckduckgo pause example', function() {
it('Search Nightwatch.js and check results', function(browser) {
browser
.url('https://duckduckgo.com')
.pause()
.waitForElementVisible('#search_form_input_homepage')
.sendKeys('#search_form_input_homepage', ['Nightwatch.js'])
.click('#search_button_homepage')
.assert.visible('.results--main')
.assert.textContains('.results--main', 'Nightwatch.js');
});
});
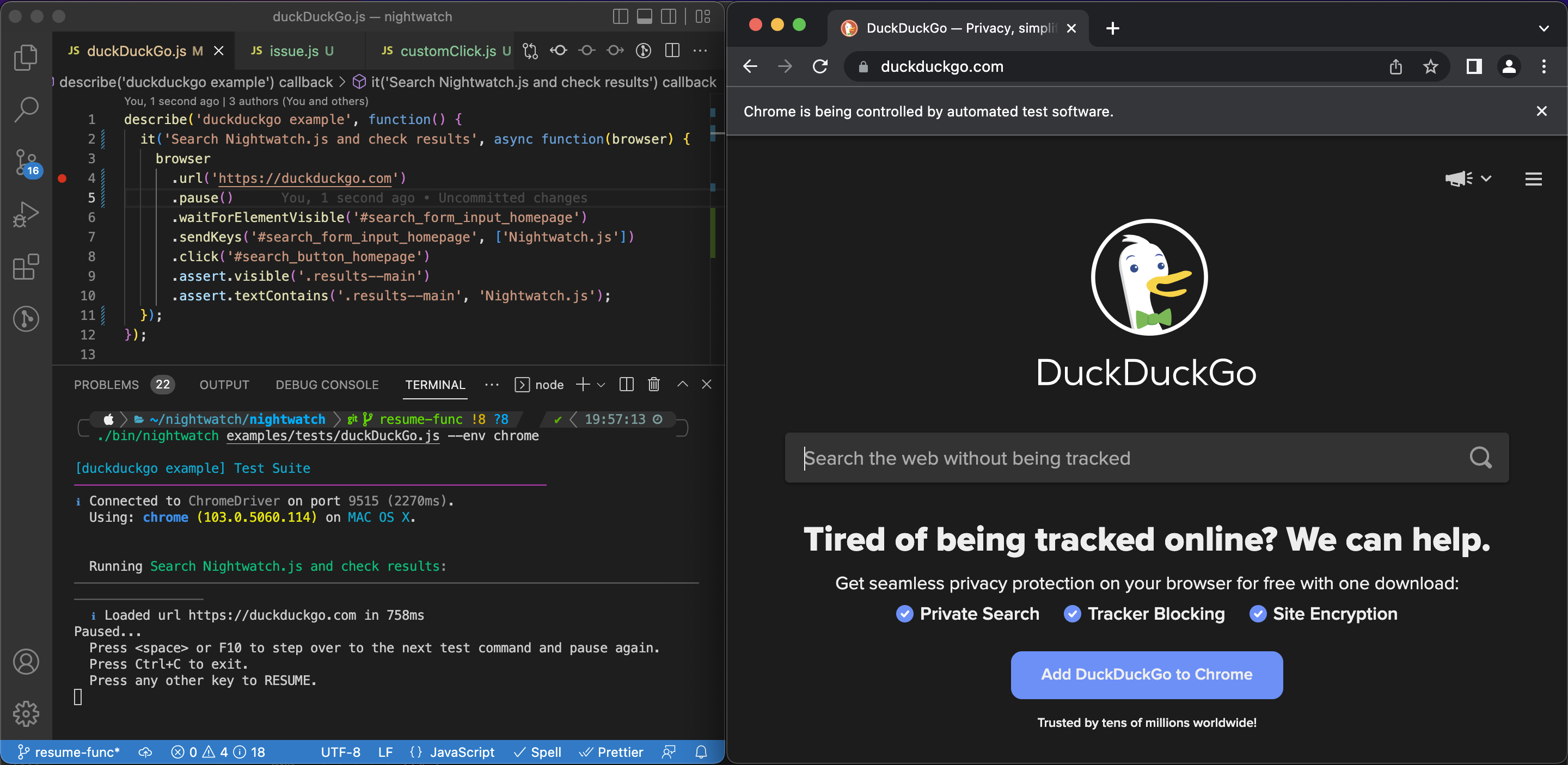