Using Ava as a test runner
Overview
Ava is a test runner for Node.js with a concise API, which embraces new language features, has detailed error output and process isolation. While Ava is mostly used to run unit tests, it can be configured with Nightwatch.js to function as an integrated test framework for performing automated end-to-end tests on web applications across all major browsers.
Configuration with Example
Step 0: install Nightwatch
Follow the guide or watch the video to install Nightwatch from scratch.
Step 1: Install Ava
npm i ava --save-dev
And make sure you have included the following script in your package.json file in order to run ava tests.
{
"scripts": {
"test": "ava"
}
}
Step 2: Configure Ava
You can configure all the CLI options in either package.json
file or can create a file ava.config.js
. Follow the guide for more details :
{
"ava": {
"files": [
"test/**/*",
"!test/exclude-files-in-this-directory",
"!**/exclude-files-with-this-name.*"
],
"match": [
// "*oo",
// "!foo"
],
"concurrency": 5,
"failFast": true,
"failWithoutAssertions": false,
"environmentVariables": {
"MY_ENVIRONMENT_VARIABLE": "some value"
},
"verbose": true,
"nodeArguments": [
"--trace-deprecation",
"--napi-modules"
]
}
}
Step 3: Nightwatch environment setup for Ava
You have to create an environment of Nightwatch to make it compatible with Ava. You need to write a _setup-nightwatch-env.js
file and make sure it has been included in your tests files :
const Nightwatch = require('nightwatch');
const createNightwatchClient = function({
headless = true,
browserName = undefined,
silent = true,
verbose = false,
output = true,
env = null,
parallel = false,
devtools = false,
debug = false,
persistGlobals = true,
configFile = './nightwatch.conf.js',
globals = {},
webdriver = {},
timeout = null,
enableGlobalApis = false,
reporter = null,
alwaysAsync = true,
desiredCapabilities = {}
} = {}) {
const client = Nightwatch.createClient({
headless,
browserName,
reporter,
env,
timeout,
parallel,
output,
devtools,
debug,
enable_global_apis: enableGlobalApis,
silent: silent && !verbose,
always_async_commands: alwaysAsync,
webdriver,
persist_globals: persistGlobals,
config: configFile,
globals,
desiredCapabilities
});
client.updateCapabilities(desiredCapabilities);
return client.launchBrowser();
};
module.exports = async (t, run) => {
global.browser = await createNightwatchClient();
try {
await run(t);
} finally {
await global.browser.end();
}
};
Nightwatch options
The default behavior of Nightwatch can be modified by supplying any of the following configuration options. Below is a list of available options and their default values.
Name | Type | Description | Default |
---|---|---|---|
headless |
Boolean | Run Nightwatch in headless mode (available in Firefox, Chrome, Edge) | true |
browserName |
String | Browser name to use; available options are: chrome, firefox, edge, safari | none |
baseUrl |
String | The base url to use for the when using .navigateTo() with relative urls. When doing component testing it needs to be set to the url running the Vite dev server. | http://localhost:3000 |
verbose |
Boolean | Enable complete Nightwatch http logs. | false |
output |
Boolean | Show Nightwatch output | true |
env |
String | Nightwatch test environment to use, from nightwatch.conf.js. Learn more about test environments in the Nightwatch docs. | none |
parallel |
Boolean | Set this to true when running tests in parallel | false |
devtools |
Boolean | Chrome only: automatically open the chrome devtools | false |
debug |
Boolean | Component testing only: pause the test execution after rendering the component | false |
autoStartSession |
Boolean | Start the Nightwatch session automatically. If this is disabled, you'll need to call jestNightwatch.launchBrowser() in your tests. | true |
persistGlobals |
Boolean | Persist the same globals object between runs or have a (deep) copy of it per each test. Learn more about test globals in the Nightwatch docs. | true |
configFile |
String | The Nightwatch config file to use. A config file will be auto-generated by default, but this allows you to change that. Learn more about the Nightwatch config in the Nightwatch docs. | ./nightwatch.conf.js |
globals |
Object | A list of globals to be used in Nightwatch. Globals are available on browser.globals. Learn more about the Nightwatch test globals in the Nightwatch docs. | none |
webdriver |
Object | A list of Webdriver related settings to configure the Nightwatch Webdriver service. Learn more about the Nightwatch webdriver settings in the Nightwatch docs. Nightwatch webdriver settings in the Nightwatch docs. | none |
timeout |
Number | Set the global timeout for assertion retries before an assertion fails. | 5000 |
enableGlobalApis |
Boolean | The Nightwatch global APIs (element(), expect()) are disable by default. | false |
desiredCapabilities |
Object | Define custom Selenium capabilities for the current session. Learn more about the specific browser driver that it is being used on the Nightwatch docs. | none |
setup() |
Function | Additional setup hook to be executed after Nightwatch has been started. | none |
teardown() |
Function | dditional teardown hook to be executed with the Nightwatch api object. | none |
Step 4: Run an example test
Consider the below example test :
const test = require('ava');
const await_nightwatch_browser = require('../../../_setup-nightwatch-env.js');
test('duckduckgo example', await_nightwatch_browser, async function(t) {
browser
.navigateTo('https://www.ecosia.org/')
.waitForElementVisible('body')
const titleContains = await browser.assert.titleContains('Ecosia');
t.is(titleContains.passed, true);
const visible = await browser.assert.visible('input[type=search]')
t.is(visible.passed, true);
t.pass();
});
To run the tests you can use the following commands :
npm test
or
npx ava
Note : Ava has different naming conventions so you should confirm that your tests are following this guide
Step 5: View the results of Ava test runner
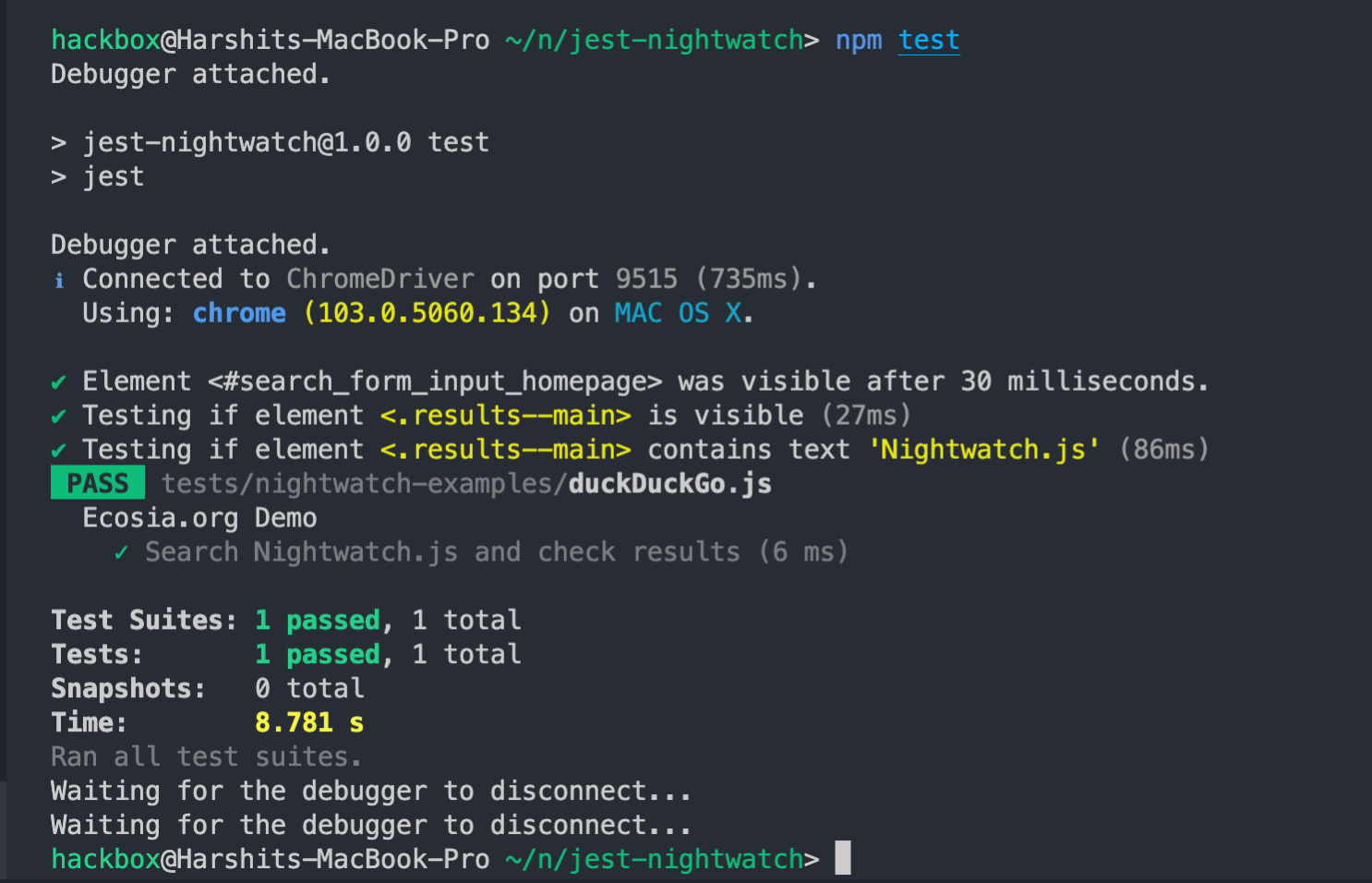
Related articles