Using Jest as a test runner
Overview
Jest is a javascript testing framework which is used to easily write unit tests whereas Nightwatch.js is an integrated test framework for performing automated end-to-end testing on web applications and websites, across all major browsers.
So to specifically use Jest and Nightwatch functionalities simultaneously, Nightwatch has introduced a new dependency jest-environment-nightwatch
.
API
global.browser
The Nightwatch browser API object. Unavailable when autoStartSession is off.
global.jestNightwatch
The Jest environment used the Nightwatch programmatic API to create the Nightwatch instance and export the browser API.
Available properties/methods:
.element(<locator>)
- use the Nightwatch .element() API to locate elements in the page;.updateCapabilities({ capabilities })
- used when autoStartSession is off in order to update the capabilities at run-time;.launchBrowser()
- used when autoStartSession is off in order to start the session and open the browser;.settings
- the Nightwatch settings object;.nightwatch_client
- the Nightwatch (internal) instance.
Configuration with Example
Step 0: install Nightwatch
Follow the guide or watch the video to install Nightwatch from scratch.
Step 1: Install Jest
npm i jest --save-dev
And make sure you have included the following script in your package.json file in order to run jest tests.
{
"scripts": {
"test": "jest"
}
}
Step 2: Install and configure the jest-environment-nightwatch package
In order to use Nightwatch with Jest, you need to install the jest-environment-nightwatch
. Install Jest and other dependencies needed for testing.
npm i jest-environment-nightwatch --save-dev
The Nightwatch Jest environment comes with default settings. But, if needed, you can tweak the Jest configuration:
{
testEnvironment: 'jest-environment-nightwatch',
testEnvironmentOptions: {
// Nightwatch related options
headless: true,
browserName: 'chrome',
baseUrl: '',
verbose: false,
output: true,
env: null,
parallel: false,
devtools: false,
debug: false,
autoStartSession: true,
persistGlobals: true,
configFile: './nightwatch.conf.js',
globals: {},
webdriver: {},
timeout: null,
enableGlobalApis: false,
alwaysAsync: true,
desiredCapabilities: {},
async setup(browser) {},
async teardown(browser) {},
},
testMatch: [
"**/tests/*.[jt]s?(x)"
],
}
You can set your test folders directory in testMatch
.
Nightwatch options
The default behavior of Nightwatch can be modified by supplying any of the following configuration options. Below is a list of available options and their default values.
Name | Type | Description | Default |
---|---|---|---|
headless |
Boolean | Run Nightwatch in headless mode (available in Firefox, Chrome, Edge) | true |
browserName |
String | Browser name to use; available options are: chrome, firefox, edge, safari | none |
baseUrl |
String | The base url to use for the when using .navigateTo() with relative urls. When doing component testing it needs to be set to the url running the Vite dev server. | http://localhost:3000 |
verbose |
Boolean | Enable complete Nightwatch http logs. | false |
output |
Boolean | Show Nightwatch output | true |
env |
String | Nightwatch test environment to use, from nightwatch.conf.js. Learn more about test environments in the Nightwatch docs. | none |
parallel |
Boolean | Set this to true when running tests in parallel | false |
devtools |
Boolean | Chrome only: automatically open the chrome devtools | false |
debug |
Boolean | Component testing only: pause the test execution after rendering the component | false |
autoStartSession |
Boolean | Start the Nightwatch session automatically. If this is disabled, you'll need to call jestNightwatch.launchBrowser() in your tests. | true |
persistGlobals |
Boolean | Persist the same globals object between runs or have a (deep) copy of it per each test. Learn more about test globals in the Nightwatch docs. | true |
configFile |
String | The Nightwatch config file to use. A config file will be auto-generated by default, but this allows you to change that. Learn more about the Nightwatch config in the Nightwatch docs. | ./nightwatch.conf.js |
globals |
Object | A list of globals to be used in Nightwatch. Globals are available on browser.globals. Learn more about the Nightwatch test globals in the Nightwatch docs. | none |
webdriver |
Object | A list of Webdriver related settings to configure the Nightwatch Webdriver service. Learn more about the Nightwatch webdriver settings in the Nightwatch docs. Nightwatch webdriver settings in the Nightwatch docs. | none |
timeout |
Number | Set the global timeout for assertion retries before an assertion fails. | 5000 |
enableGlobalApis |
Boolean | The Nightwatch global APIs (element(), expect()) are disable by default. | false |
desiredCapabilities |
Object | Define custom Selenium capabilities for the current session. Learn more about the specific browser driver that it is being used on the Nightwatch docs. | none |
setup() |
Function | Additional setup hook to be executed after Nightwatch has been started. | none |
teardown() |
Function | dditional teardown hook to be executed with the Nightwatch api object. | none |
Step 3: Run an example test
Consider the below example test :
describe('duckduckgo example', function() {
it('Search Nightwatch.js and check results', function(browser) {
browser
.navigateTo('https://duckduckgo.com')
.waitForElementVisible('#search_form_input_homepage')
.sendKeys('#search_form_input_homepage', ['Nightwatch.js'])
.click('#search_button_homepage')
.assert.visible('.results--main')
.assert.textContains('.results--main', 'Nightwatch.js');
});
});
Note : Jest has different naming conventions for hooks so you should confirm that your tests are following this guide.*
Step 4: View the results of Jest test runner
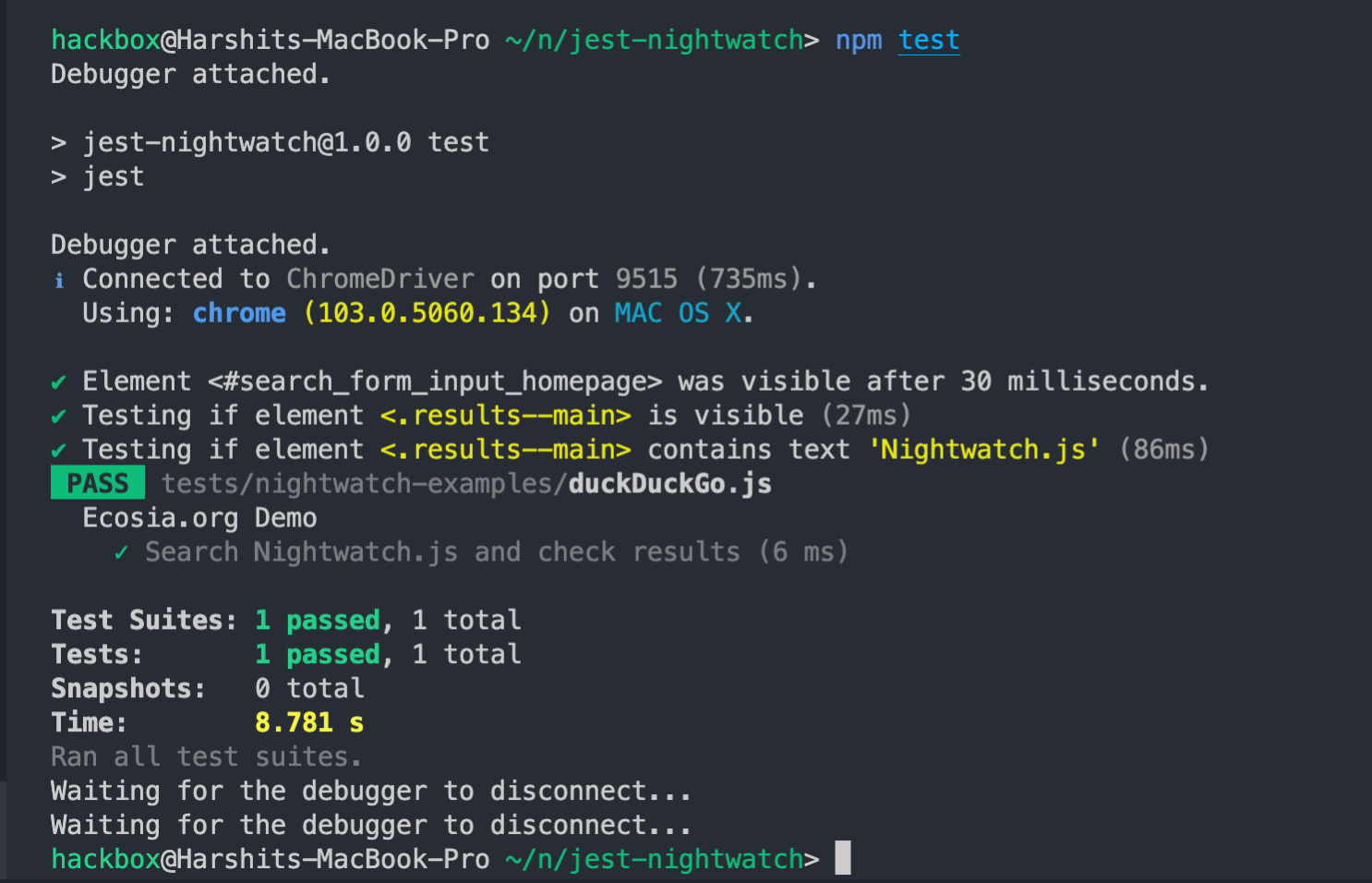